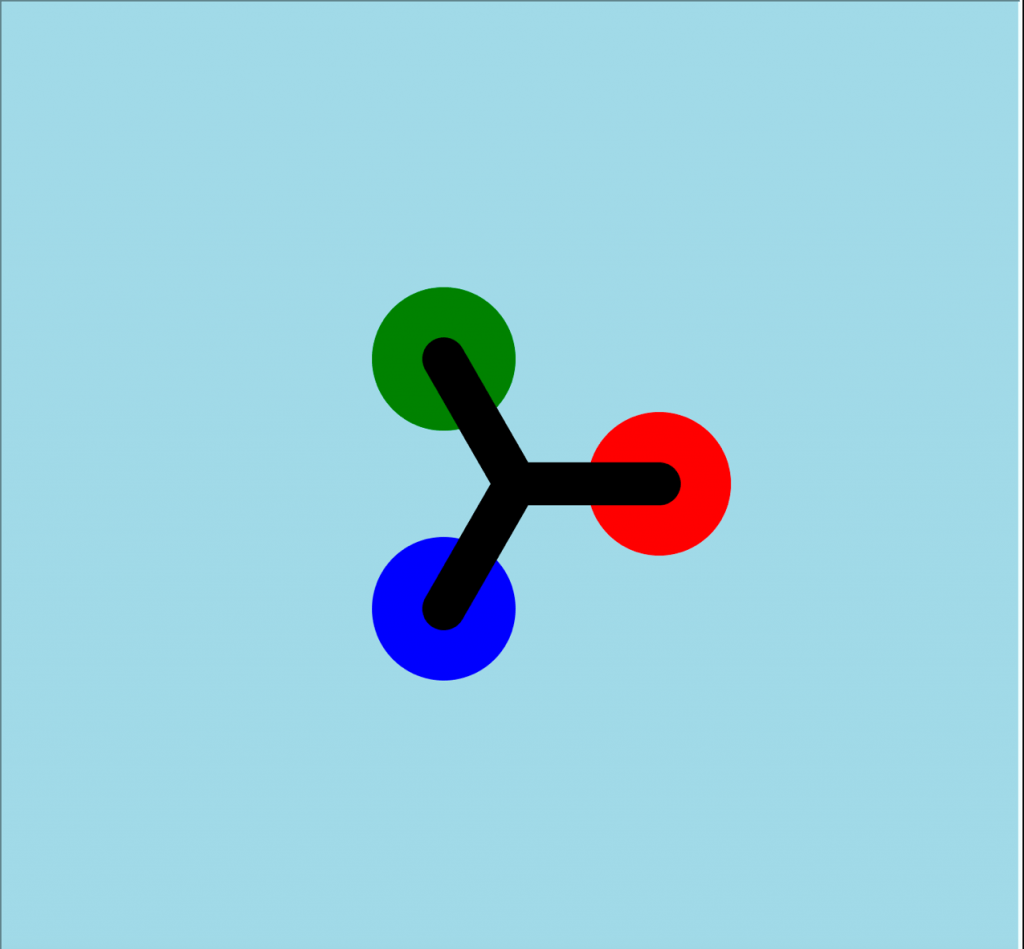
There are plenty of games in python. One of them is the Fidget Spinner. You can create Fidget Spinner using a python tutorial. The Fidget Spinner is an awesome game to play in your free time. It uses turtle graphics and will give you a flavor of different functions of the turtle module. It also makes use of a dictionary. Also, the game code gives you an idea of event-handling in python. You can watch how Anvita, a student at Skoolofcode, has created the Fidget Spinner Using Python Coding for kids tutorial.
So, let’s get started.
Fidget Spinner Using Python
Step 1:
For creating Fidget Spinner using Python, the first step is to import the turtle module and set up the screen. See the following lines of code:
1 import turtle
2 scr = turtle.Screen()
3 scr.bgcolor("lightblue")
The second line of code creates a screen object scr. The third line of code sets the background color to light blue. You can type any color of your choice(but should be a valid color choice).
Step 2:
Next step is to create a pen turtle object using the Turtle() function. Line 4 tells that. In line 5, we set the pen color of the turtle to black. In line 6, we set the size of the pen to 30.
4 turt = turtle.Turtle()
5 turt.pencolor("black")
6 turt.pensize(30)
Step 3:
Since we want to create three circles of different colors, it’s useful to create a list of these colors. Line 7 tells us that. Here, we can see the strength of Python as a language. We can store different colors in one list.
7 col = ["red","blue","green"]
8 state = {'turn':0}
In line 8, we create a dictionary state with only one key-value pair. The key is ‘turn’ and its value is initialized to 0. The key ‘turn’ will decide the turning angle of the spinner as you will observe in the rest of the code. This is the reason it’s amazing to create Fidget Spinner using Python.
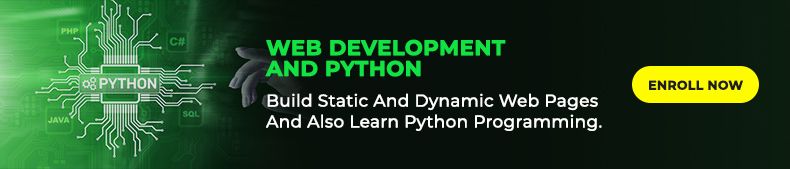
Step 4:
We need to define three functions.
- spinner(): This function is defined to create the fidget spinner itself.
- animate(): This function is needed to animate the spinner.
- flick(): This function is defined to flick the spinner, every time we press the space key on the keyboard.
spinner(): First we need to create a static spinner image. We need the dot() function to create the three circles.
Line 10: using a for loop to run the block of code 3 times.
Line 11: Move the turtle forward by 100 pixels.
Line 12: Draw a dot using the dot function with a radius of 100 pixels and a color from the list.
Line 13: Go backward by 100 pixels.
Line 14: Turn right by 120 degrees and repeat the last 3 steps.
Add the following lines of code. Call the spinner() function at the end of your program and run your code. You will be able to see a static fidget spinner image.
9 def spinner():
10 turt.clear()
11 angle = state['turn']/10
12 turt.right(angle)
13 for i in range(3):
14 turt.forward(100)
15 turt.dot(100,col[i])
16 turt.backward(100)
17 turt.right(120)
18. turtle.update()
The function code is not yet complete. We will first define the rest two functions and then come back again on the spinner() function code.
Step 5:
In this step, we will learn how to define the flick() function. You will be amazed to see that this function has just used scratch coding of one-line code.
19 def flick():
20 state['turn'] += 10
Each time you call a flick() function, it increases the value of key ‘turn’ by 10. This helps in increasing the turning angle of the spinner each time you flick.
Step 6:
Now let’s talk about the animate() function.
21 def animate():
22 if state['turn']>0:
23 state['turn'] -= 1
24 spinner()
25 turtle.ontimer(animate,20)
Line 17 gives the function definition.
Line 18 and 19 checks a condition that if ‘turn’ is greater than 0, then reduce turn by -1.
Line 20 calls the spinner() function.
Line 21 calls the ontimer() function of the turtle module which is used to call the animate() function after every 20 seconds.
Step 7:
We will add the following lines of code in the spinner() function definition to take into account the turning angle.
10 turt.clear()
11 angle = state['turn']/10
12 turt.right(angle)
18. turtle.update()
Line 10 and 18 are added to clear the previous image created by the turtle and update with the new image.
Line 11 sets the angle variable to the value of ‘turn’ divided by 10.
Line 12 turns the spinner by angle degrees.
Step 8:
In this step, the following code is added to the main function.
26. scr.tracer(False)
27. turtle.onkey(flick,"space")
28. turtle.listen()
29. animate()
30. scr.mainloop()
Line 26 sets the tracer to False which means that we will not see each line being traced. Line 27 calls the turtle.onkey() function which itself calls the function flick() when the space key is pressed.
Line 28 calls listen() function which makes it possible to listen to the keypress events. Line 29 calls the animate() function. Line 30 calls the mainloop() function which is an infinite loop that keeps the screen working till the user closes it.
SOURCE CODE:
1. import turtle
2. scr = turtle.Screen()
3. scr.bgcolor("lightblue")
4. turt = turtle.Turtle()
5. turt.pencolor("black")
6. turt.pensize(30)
7. col = ["red","blue","green"]
8. state = {'turn':0}
9. def spinner():
10. turt.clear()
11. angle = state['turn']/10
12. turt.right(angle)
13. for i in range(3):
14. turt.forward(100)
15. turt.dot(100,col[i])
16. turt.backward(100)
17. turt.right(120)
18. turtle.update()
19. def flick():
20. state['turn'] += 10
21. def animate():
22. if state['turn']>0:
23. state['turn'] -= 1
24. spinner()
25. turtle.ontimer(animate,20)
26. scr.tracer(False)
27. turtle.onkey(flick,"space")
28. turtle.listen()
29. animate()
30. scr.mainloop()
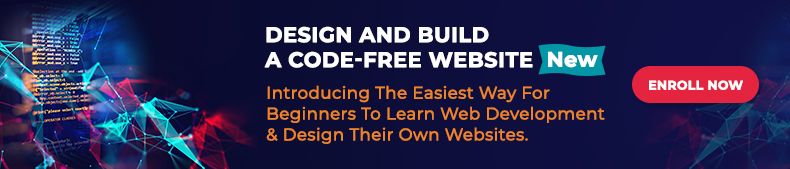
I hope that the above code is useful and will help you in creating a Fidget Spinner using Python. You can also have a glimpse of a similar video presentation by Aneek and Aneesh who applied for coding for 3rd graders. At SkoolofCode, we offer project-based learning modules where students use live code to find logical and inventive solutions to problems. So, why wait and Book a FREE trial class today.