Race the turtles using Python!
Aaryaman is a student at Skoolofcode. In Skoolofcode he is learning how to code in Python using some fun projects. He got a chance to build a program called Turtle Race game using which he learned the concepts of Loops and User-Defined Functions in Python.
What is Turtle Race Game
In this game, there are 4 turtles and all are moving with a different random number of steps. The objective of the game is to find which turtle reaches the Finish line first so we can declare it as a winner!
The logic for the Turtle Race game
Aaryaman is excited to show you how he did it. Want to see the Turtle race game? Before we start building it lets understand the logic first.
LOGIC-
- There is a race track with 10 lines. 1st Line is the Starting Line and the 10th line is the Finish Line.
- There are 4 turtles with different colors placed before the Starting Line initially.
- Every turtle will take a random number of steps to move forward on the race track continuously.
- On the other end of the race track is the Finish Line. The first one to reach the Finish Line is declared as the winner and the game stops.
Understood the logic? Then let’s get started!
Prerequisites for building the Turtle Race game
Aaryaman built this Turtle Race Program using Python3 and Turtle module of python. Turtle module in python helps to learn computer coding for kids through fun projects. The turtle module is shipped with python. So, we can just start building the game by creating a new python file.
If you don’t have python installed on your system, you can install it from https://www.python.org/ Also, to write the code you can simply use python IDLE which comes along with python and is a great tool for writing and executing the code or you can use IDE like PyCharm or Visual Studio Code.
Building the Turtle Race game
Now let’s code the logic we discussed above!
Import the modules and set up turtle screen
The 1st line of our Race the Turtles game will be to import the required modules-
import random, turtle
Next, let’s create the turtle screen using the Screen class of turtle module-
myscreen= turtle.Screen()
Let’s do a quick setup of the screen by setting its background color to light blue (or any color of your choice) and setting its width and height to full size and giving a title to it-
myscreen.bgcolor('light blue')
myscreen.setup(1.0,1.0)
myscreen.title('Turtle Race Game')
Execute the python file and see how your turtle screen looks.
The screen got closed of its own?
Add turtle.done()
at the end of the code so the screen is not closed automatically.
So, your screen should look like this-
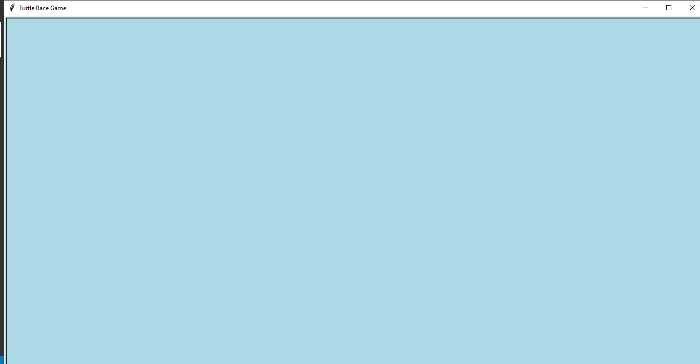
Create a pen
We are done with the setup! Now we need a pen to draw a race track. Let’s create one using Turtle class of turtle module-
pen=turtle.Turtle()
pen.speed(0) # so it moves with fastest speed
Build the race track
We need to place the pen from where we want to start drawing the race track.
Let’s consider we want the Starting Line to be drawn at x position -200 and y position 200.
pen.penup() #pen goes up as we don't want to draw on the line.
pen.goto(-200,200) #this is x and y position from center of the screen
pen.pendown() # pen placed down again
So ready to draw race track?
So first let’s create the 10 lines that will be part of our race track.
We can use for loop for 10 iterations-
for i in range(1,11): # this will run from 1 to 10
Now we can just change direction of pen in downward direction and start drawing the line-
for i in range(1,11): # this will run from 1 to 10
pen.setheading(-90) #this will point pen in downward direction
pen.forward(250) #draw a line of 250 pixels length.
Let’s consider all lines to be 50 pixels apart. So we need to go back up and then move 50 pixels ahead in right direction.
for i in range(1,11): # this will run from 1 to 10
pen.setheading(-90) #this will point pen in downward direction
pen.forward(250) #draw a line of 250 pixels length.
pen.back(250) #goes back
pen.penup() #pen goes up as we don't want to draw on line
pen.setheading(0) #pen points in right direction
pen.forward(50) #space of 50 pixels between each line of
pen.down() #pen down again
Our race track is almost ready!
Mark the race tracks
We can also mark each of the race tracks by simply using the write()
function-
for i in range(1,11): # this will run from 1 to 10
pen.write(i) #writing the race track number before each line
pen.setheading(-90) #this will point pen in downward direction
pen.forward(250) #draw a line of 250 pixels length.
pen.back(250) #goes back
pen.penup() #pen goes up as we don't want to draw on line
pen.setheading(0) #pen points in right direction
pen.forward(50) #space of 50 pixes between each line
pen.down() #pen down again
We can also mark 10th line as the finish line by checking the value of iterating variable using if condition statement-
for i in range(1,11): # this will run from 1 to 10
pen.write(i) #writing the race track number before each line
pen.setheading(-90) #this will point pen in downward direction
pen.forward(250) #draw a line of 250 pixels length.
if i==10: # this if condition will be true only if the iterating variable is 10
pen.write(" FINISH")
pen.back(250) #goes back
pen.penup() #pen goes up as we don't want to draw on line
pen.setheading(0) #pen points in right direction
pen.forward(50) #space of 50 pixes between each line
pen.down() #pen down again
Note- You can use an optional font argument in write() function to change text style on turtle screen as follows-
pen.write(i,font=('Arial',10))
pen.write(" FINISH",font=('Arial',14))
We did it! Race Track of 10 lines is ready!
Here is how our race track looks like now-
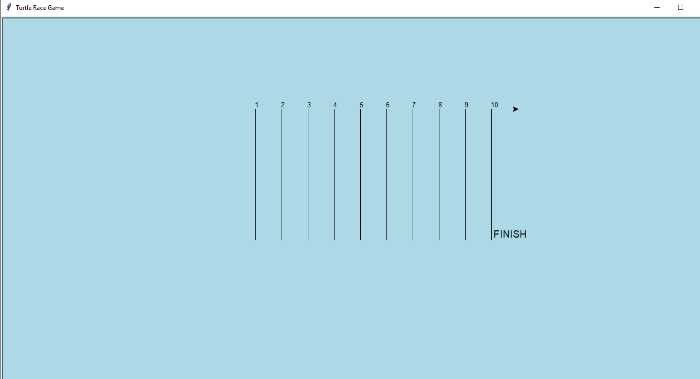
Define the finish line position
Quick math- 1st line is at x position -200, all 10 lines are 50 pixels apart each so 10th line will be at x position 250
So let’s create a variable to store the x position of Finish Line so later we can check which turtle reaches it first.
finishLineX=250
Create turtle players
Now to create 4 turtle players we can define a function let’s say createTurtlePlayer() function. We will call this function 4 times passing different colors and x and y positions for each of the turtle players.
Let’s first define the function-
def createTurtlePlayer(color, startx, starty):
In the function we can create turtle using Turtle class, set the color, set it shape to look like turtle and place it at the mentioned location.
def createTurtlePlayer(color, startx, starty):
player=turtle.Turtle()
player.color(color) # set the color of turtle
player.shape("turtle") #set the shape as turtle
player.penup() #pen moves up
player.goto(startx, starty) #place player at mentioned position on race track.
player.pendown() #pen placed down
return player #returns the turtle player object.
Now let’s create 4 turtle players by calling this function 4 times with different colors and positions.
p1=createTurtlePlayer('red',-210,150) #red colored turtle at x position before 1st Line and y position 150
p2=createTurtlePlayer('blue',-210,100) #blue colored turtle at x position before 1st Line and y position 100
p3=createTurtlePlayer('orange',-210,50) #orange colored turtle at x position before 1st Line and y position 50
p4=createTurtlePlayer('green',-210,0) #green colored turtle at x position before 1st Line and y position 0
See how all 4 turtles are placed on the starting line-
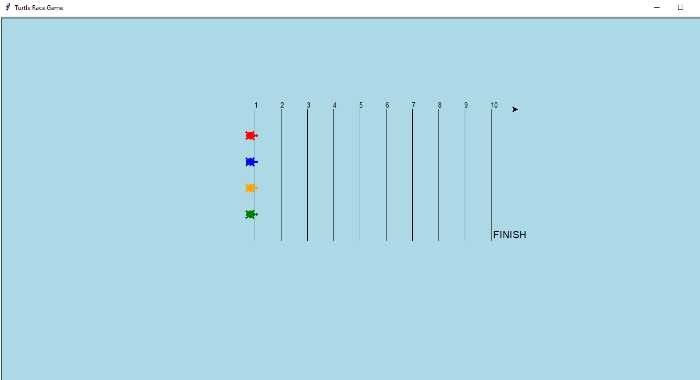
Race time!
All turtles created! Now we can race them!
So let the race begin!
We need to move all the turtle players continuously so we can use a while loop-
while True:
Now we need to move each of the turtles forward with random speed so we can use randint() function from random module to generate random number between 5 and 10 ( we will set 5 as minimum speed and 10 as the maximum speed)-
while True:
p1.forward(random.randint(5,10))
p2.forward(random.randint(5,10))
p3.forward(random.randint(5,10))
p4.forward(random.randint(5,10))
So the turtle players are moving continuously. What next?
Declare the winner
Now we need to check if any turtle player reaches the Finish Line first so we can declare it as the winner.
We can check this using a pos() function of turtle player to get the x position and compare it with our finishLineX variable which we already created.
while True:
p1.forward(random.randint(5,10))
if p1.pos()[0]>=finishLineX:
p1.write('I won the race!!',font=('Arial',30))
p2.forward(random.randint(5,10))
if p2.pos()[0]>=finishLineX:
p2.write('I won the race!!',font=('Arial',30))
p3.forward(random.randint(5,10))
if p3.pos()[0]>=finishLineX:
p3.write('I won the race!!',font=('Arial',30))
p4.forward(random.randint(5,10))
if p4.pos()[0]>=finishLineX:
p4.write('I won the race!!',font=('Arial',30))
So we got the winner!!
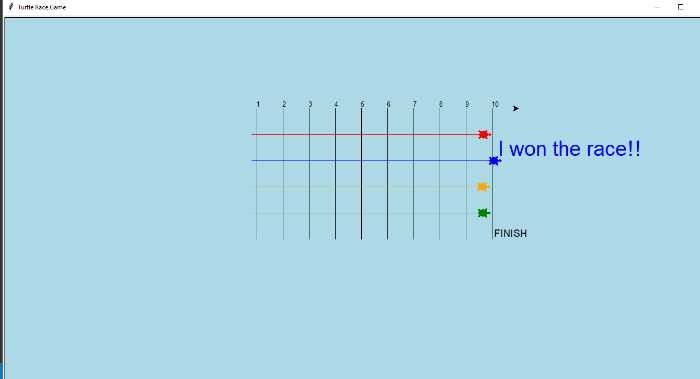
Race ends!
If we get the winner then we can stop the game using break statement-
while True:
p1.forward(random.randint(5,10))
if p1.pos()[0]>=finishLineX:
p1.write('I won the race!!',font=('Arial',30))
break
p2.forward(random.randint(5,10))
if p2.pos()[0]>=finishLineX:
p2.write('I won the race!!',font=('Arial',30))
break
p3.forward(random.randint(5,10))
if p3.pos()[0]>=finishLineX:
p3.write('I won the race!!',font=('Arial',30))
break
p4.forward(random.randint(5,10))
if p4.pos()[0]>=finishLineX:
p4.write('I won the race!!',font=('Arial',30))
break
There we go! Our race is over.
Happy Coding! Hope you had fun building the game.
Online coding course with a professional instructor? The instructors at SkoolofCode have years of expertise teaching computer programming online and hold degrees in computer science or engineering. So, why wait and Book a FREE trial class today.
Final Source Code for Turtle Race game-
Here is the whole source code-
import random, turtle
myscreen= turtle.Screen()
myscreen.bgcolor('light blue')
myscreen.setup(1.0,1.0)
myscreen.title('Turtle Race Game')
pen=turtle.Turtle()
pen.speed(0) # so it moves with fastest speed
pen.penup() #pen goes up as we don't want to draw on line.
pen.goto(-200,200) #this is x and y position from center of the screen
pen.pendown() # pen placed down again
for i in range(1,11): # this will run from 1 to 10
pen.write(i,font=('Arial',10)) #writing the race track number before each line
pen.setheading(-90) #this will point pen in downward direction
pen.forward(250) #draw a line of 250 pixels length.
if i==10: # this if condition will be true only if the iterating variable is 10
pen.write(" FINISH",font=('Arial',14))
pen.back(250) #goes back
pen.penup() #pen goes up as we don't want to draw on line
pen.setheading(0) #pen points in right direction
pen.forward(50) #space of 50 pixes between each line
pen.down() #pen down again
finishLineX=250
def createTurtlePlayer(color, startx, starty):
player=turtle.Turtle()
player.color(color) # set the color of turtle
player.shape("turtle") #set the shape as turtle
player.penup() #pen moves up
player.goto(startx, starty) #place player at mentioned position on race track.
player.pendown() #pen placed down
return player #returns the turtle player object.
p1=createTurtlePlayer('red',-210,150) #red colored turtle at x position before 1st Line and y position 150
p2=createTurtlePlayer('blue',-210,100) #blue colored turtle at x position before 1st Line and y position 100
p3=createTurtlePlayer('orange',-210,50) #orange colored turtle at x position before 1st Line and y position 50
p4=createTurtlePlayer('green',-210,0) #green colored turtle at x position before 1st Line and y position 0
while True:
p1.forward(random.randint(5,10))
if p1.pos()[0]>=finishLineX:
p1.write(' I won the race!!',font=('Arial',30))
break
p2.forward(random.randint(5,10))
if p2.pos()[0]>=finishLineX:
p2.write(' I won the race!!',font=('Arial',30))
break
p3.forward(random.randint(5,10))
if p3.pos()[0]>=finishLineX:
p3.write(' I won the race!!',font=('Arial',30))
break
p4.forward(random.randint(5,10))
if p4.pos()[0]>=finishLineX:
p4.write(' I won the race!!',font=('Arial',30))
break
turtle.done()