Want to build a number guessing game and not sure how to generate a random number? Or a program that generates a random sequence of words or colors? Well, generating random numbers in Python with random module is easy.
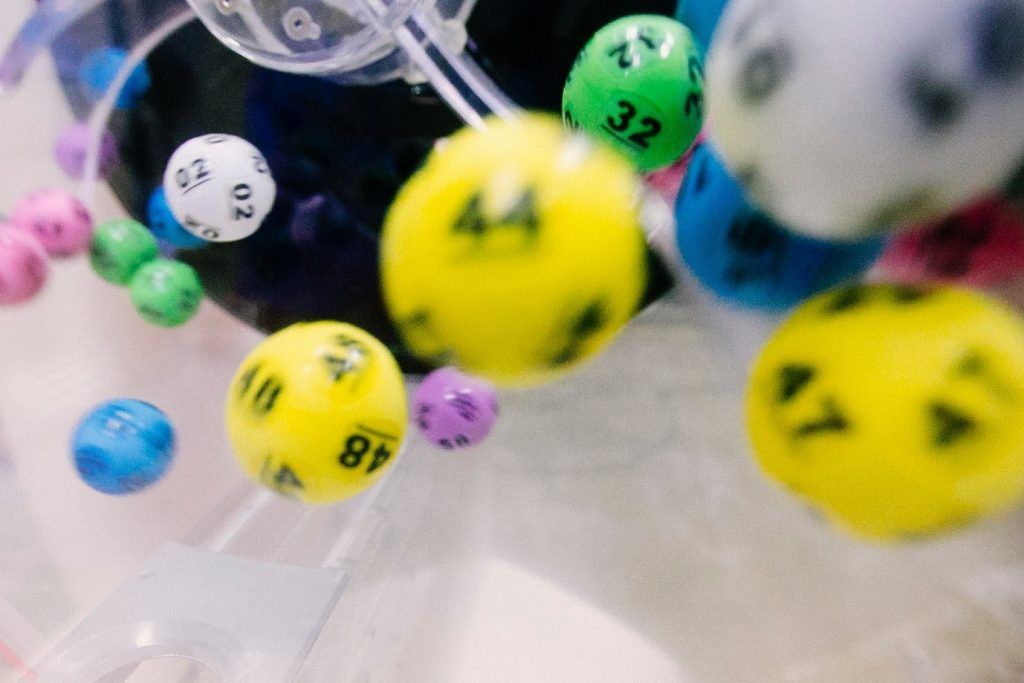
Python has a standard module, random, to generate random numbers and sequences. The random module has many functions which help us to generate random numbers. Let’s see how to generate random numbers in python with a few of the functions from the random module.
Generating random integers
In role-playing games such as the Turtle Race game or Rock Paper Scissors Shoot, we need random integers. Sophia a student at Skoolofcode, built her Guess the Number game by generating random integers. Simulating the Roll, a dice program also needs a random integer.
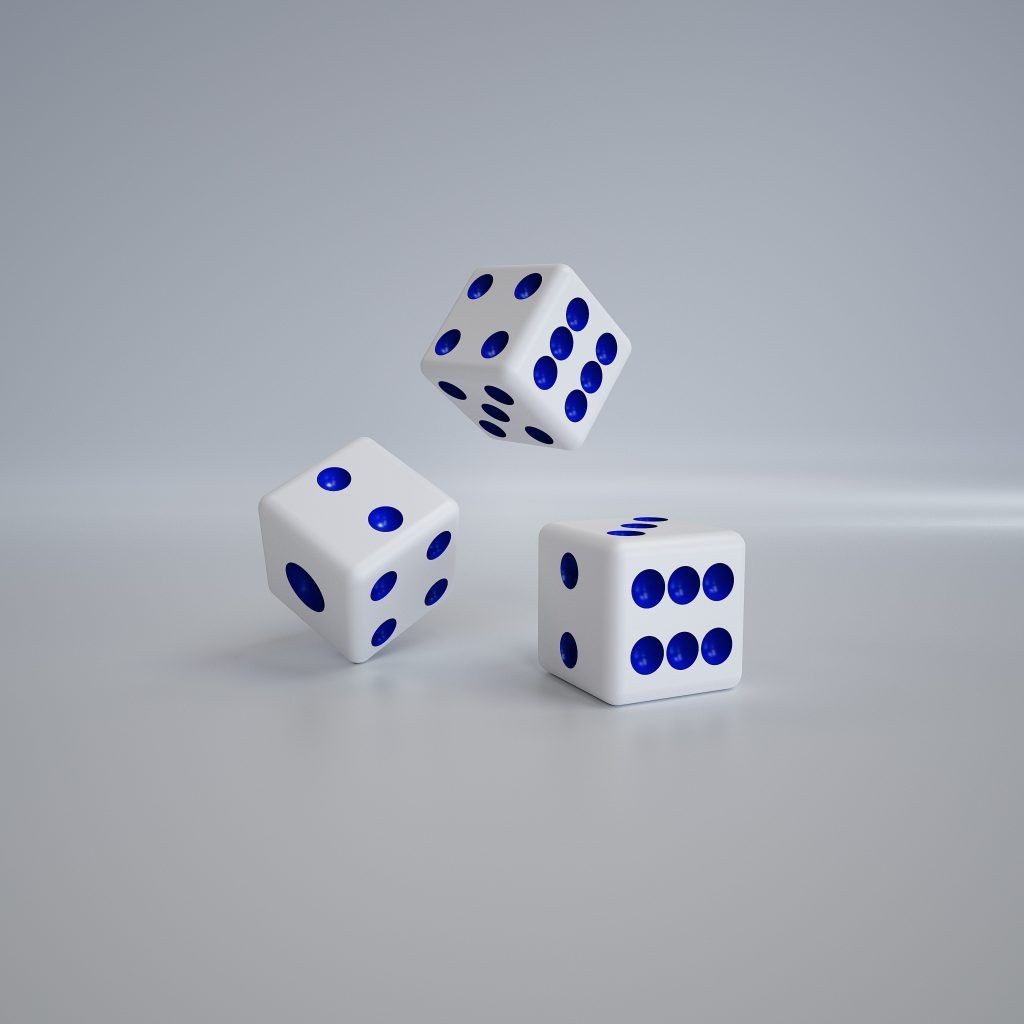
random.randrange(start,stop,step)-
This function returns random integer between the range you specify. If you don’t specify the start value, it will be set to 0. The stop value specifies the end of the range. Step specifies the increment to added to the previous number. Default step value is 1.
Let’s see a few examples-
random.randrange(10)
This prints a random number between 0 to 10 (exclusive).
random.randrange(1,5)
This will print either 1 or 2 or 3 or 4.
random.randrange(6,0,-1)
This will print any random integer from numbers 6,5,4,3,2,1.
random.randrange(20,10,-2)
Will print any of the random numbers from 12 or 14 or 16 or 18 or 20.
random.randint(start,stop)
This function generates a random integer between the range specified. Both the start and the stop values are mandatory and the stop value is inclusive.
An example-
random.randint(5,10)
This prints a random number between 5 to 10 (inclusive).
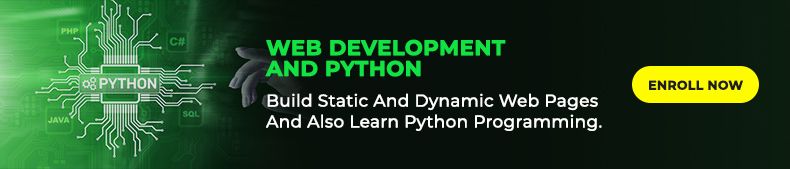
Generating random floating-point numbers
We can also generate random floating-point numbers.
random.uniform(start,stop)
This function returns a random real number between the range specified (inclusive). Both the start and the stop values are mandatory.
Example-
print(random.uniform(1,10))
Will print a random float number between 1 and 10 (inclusive).
random.random()
This function returns a random floating-point number between 0 to 1 (inclusive).
Generating random sequences
We can also use the random module to add randomness to any sequence. For example, shuffling a list of words or choosing a random color from a tuple of colors. Let’s see how.
random.choice(sequence)
This function returns random elements from the sequence. This function is most often used to get random words in-game building projects. See how Ariv-a student at Skoolofcode, used this function in his Hangman Game project.
Examples-
words = [‘cat’, ‘dog’, ‘pig’]
print(random.choice(words))
Will print a random word from the list of words.
colors=(“black”, “blue”, “orange”, “red”, “pink”, “white”, “yellow”, “green”)
chosencolor=random.choice(colors)
The variable chosencolor will hold a random color (string) from the tuple of colors.
print(random.choice(“Skoolofcode”))
Will print a random character from the string “Skoolofcode”.
random.shuffle(sequence)
This shuffles the sequence elements in random order.
Example-
words = [‘cat’, ‘dog’, ‘pig’]
random.shuffle(words)
This will shuffle words into any random order.
random.sample(sequence, k)
This function returns random elements from the sequence (up to k elements). The parameter k is optional. If not specified, it will return all the available elements from the sequence in random order.
Example-
colors = (“black”, “blue”, “orange”, “red”, “pink”, “white”, “yellow”, “green”)
print(random.sample(colors,4))
The function call above will print 4 random colors from the tuple of color values.
print(random.sample(words,5))
This will print 5 random words from the list of words.
Initialising the random number generator
Sometimes we may want to generate a particular sequence or number with some randomness. Let’s see how to do that in Python.
random.seed([x])
Seeds play an important role in Python’s pseudorandom number generator algorithm. This function sets an initial seed value for the pseudo-random number generator. If you don’t pass any parameter to this, the seed will be set to the current timestamp or os.getpid().
Example-
random.seed()
Sets the seed to current timestamp.
Another example-
random.seed(10)
print(random.random())
This seeds the random number generator using 10 as the seed, so the pseudo-random number will always be the same.
Another example-
random.seed(5)
print(random.choice(“Skoolofcode”))
This will always print a character ‘d’ from string Skoolofcode.
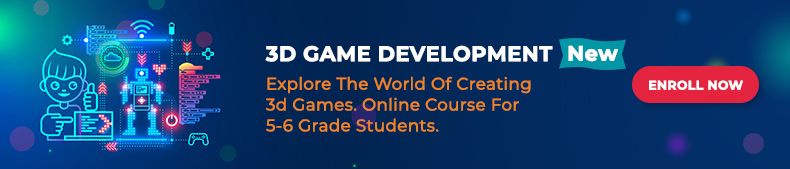
At SkoolofCode, we offer coding classes for kids that help them to understand basics of Python and build their projects. So, why wait and Book a FREE trial class today.
That’s it for this article. I hope you learned something new. But that’s not all. Python is an amazing programming tutorial online! Like any other high-level programming language, Python has all the features that make it a powerful language for game programming. There are many other functions in the random module. Refer to the official documentation of the random module in Python to learn more. Happy coding