Coding is a very important skill as it enhances logical thinking. Coding for kids isn’t that hard and can be rewarding too! At Skoolofcode, courses are designed to teach programming concepts through fun projects. These projects are both engaging and challenging. Sachintan built the Rock Paper Scissors game while learning Python course at Skoolofcode.
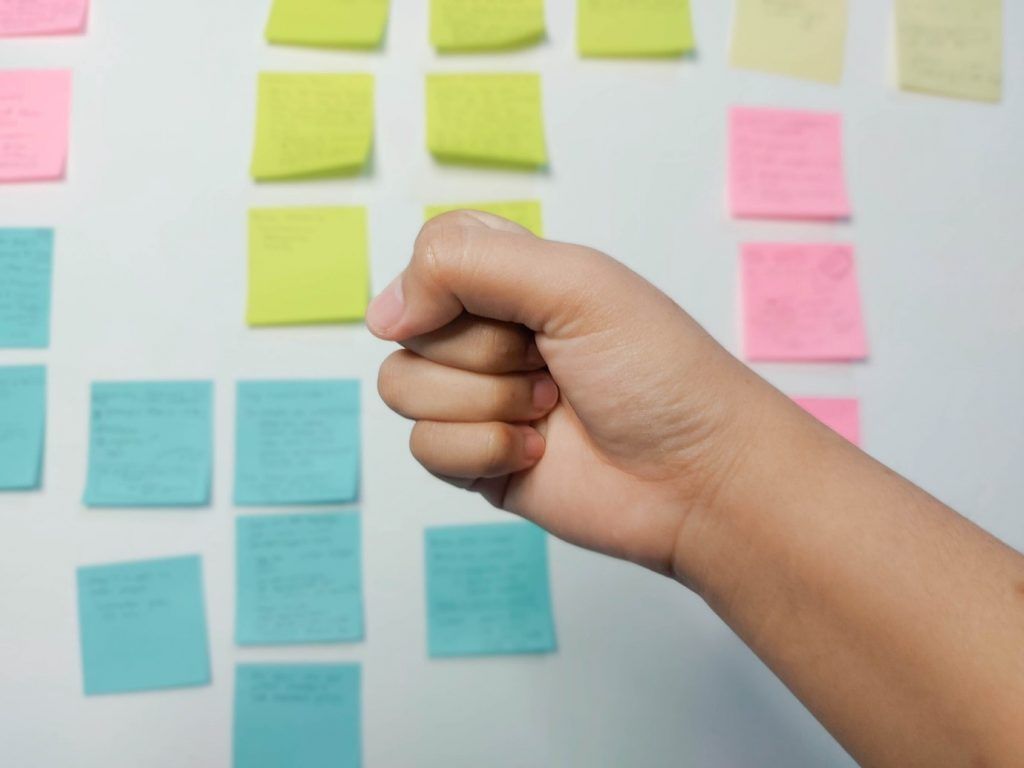
In this article, we will see how Sachintan built this Rock Paper Scissors Game using Python.
Rock Paper Scissors game is the simplest Coding Project you can do with your kids. It’s also great coding classes for kids Concepts like Loops and Conditional Statements.
What is Rock Paper Scissors game?
Rock Paper Scissors is a game of chance. In this game two players signify rock, paper, or scissors with their hands at the same time and then reveal them. We determine the winner of the match by the following rules:
- Rock blunts Scissors (Rock wins over Scissors)
- Scissors cuts Paper (Scissor wins over Paper)
- Paper covers Rock (Paper wins over Rock)
- A tie results if both players choose the same option; in this case, players play another round to break the tie.
Algorithm for Rock Paper Scissor game
We can build this game such as a user can play this game against the computer.
Let’s see the algorithm for the game step by step-
- Make a list of 3 options- Rock, Paper, Scissor
- Ask the player’s name so we can display it on the scoreboard
- Initialize the scores of player and computer to 0 and number of rounds to 0.
- Set the gameOn flag to True
- While gameOn flag is True repeat steps 6 to 12
- Randomly generate one of the options from the list as ComputerOption
- Let the player choose any option from Rock, Paper, Scissor as PlayerOption.
- Increase number of rounds by 1
- If both player and computer, choose the same option then the current round ends in a draw. Else proceed to step 10
- Determine the winner based on the rules and increase the score of the winner by 1.
- Display the updated score.
- If the number of rounds is greater than 5 then set the gameOn flag to false and end the game.
- Choose and display the final winner based on the total score.
Prerequisite
You will need Python installed on your computer. You can install python from https://www.python.org
You can write this program in Python IDLE which comes along with Python. Or you can use any other IDE like Visual Studio Code, PyCharm, etc.
Coding the game
Time for coding! So we understood the algorithm. Now let’s code the game.
We are going to let the computer choose the random option. So we will have to import the random module.
import random
Now, we make a list of options-
options=['Rock','Paper','Scissor']
Let’s ask the user his name so we can display his name on the scoreboard-
name=input("Enter your name :")
Now, initialize the scores and number of rounds-
ComputerScore=0
PlayerScore=0
NumberOfRounds=0
We will set gameOn to True to start the game and False when it finishes.
gameOn=True
Let’s print a welcome message for the player-
print(f"Welcome {name.title()}")
Note-We use title() function to convert the first letter of each of the words in the name to upper case.
So all set? Let’s play the game!
while gameOn:
ComputerOption=random.choice(options)
Here we let the computer choose a random option from the list using the choice() function of the random module.
Now Let the player choose his option-
PlayerOption=input("Enter Rock/ Paper/ Scissor :").title()
We can also display options chosen by both-
print(f"Computer option :{ComputerOption}")
print(f"{name.title()} option :{PlayerOption}")
Increase the number of rounds by 1
NumberOfRounds += 1
Compare the options chosen by computer and player
If both the computer and the player choose the same option, then declare it as a Tie.
if ComputerOption==PlayerOption:
print('Tie')
Otherwise determine the winner based on the game rules
elif (ComputerOption=='Rock' and PlayerOption == 'Scissor') or (ComputerOption=='Scissor' and PlayerOption=='Paper') or (ComputerOption=='Paper' and ComputerOption=='Rock'):
print("Computer wins")
ComputerScore += 1
elif (PlayerOption=='Rock' and ComputerOption == 'Scissor') or (PlayerOption=='Scissor' and ComputerOption=='Paper') or (PlayerOption=='Paper' and ComputerOption=='Rock'):
print(f"{name.title()} wins")
PlayerScore += 1
else:
print("Choose a valid option to play this game.")
Note that we increased the score of the winner by one.
We also made sure to display a message to the player when he chooses any unexpected option.
Let’s display the score in each round-
print("-------------------------")
print("")
print(f"Round No: {NumberOfRounds}")
print("------ Score Board ------")
print(f"{name.title()}: {PlayerScore} | Computer: {ComputerScore}")
print("===============================")
print("")
We need to make sure to end the game if 5 rounds are over.
if NumberOfRounds==5:
gameOn=False
break
Here, we check whether the number of rounds is greater than 5. If true, set the gameOn flag to False and break from the while loop. So all rounds over! Let’s display the final winner based on their total scores-
if PlayerScore==ComputerScore:
print("Draw!!")
elif PlayerScore>ComputerScore:
print(f"Congrats {name.title()}, You won the game!!")
else:
print(f"Oops Computer won the game!! Better luck next time {name.title()}!")
So we got the winner!! Hope you enjoyed building the game. For building more such exciting games Book a FREE trial class today and explore the world of game creation.
Source Code
Here is the final source code of the whole game-
import random
options=['Rock','Paper','Scissor']
name=input("Enter your name :")
ComputerScore=0
PlayerScore=0
NumberOfRounds=0
gameOn=True
print(f"Welcome {name.title()}")
while NumberOfRounds<5:
ComputerOption=random.choice(options)
PlayerOption=input("Enter Rock/ Paper/ Scissor :").title()
print(f"Computer option :{ComputerOption}")
print(f"{name.title()} option :{PlayerOption}")
NumberOfRounds += 1
if ComputerOption==PlayerOption:
print('Tie')
elif (ComputerOption=='Rock' and PlayerOption == 'Scissor') or (ComputerOption=='Scissor' and PlayerOption=='Paper') or (ComputerOption=='Paper' and ComputerOption=='Rock'):
print("Computer wins")
ComputerScore += 1
elif (PlayerOption=='Rock' and ComputerOption == 'Scissor') or (PlayerOption=='Scissor' and ComputerOption=='Paper') or (PlayerOption=='Paper' and ComputerOption=='Rock'):
print(f"{name.title()} wins")
PlayerScore += 1
else:
print("Choose a valid option to play this game.")
print("-------------------------")
print("")
print(f"Round No: {NumberOfRounds}")
print("------ Score Board ------")
print(f"{name.title()}: {PlayerScore} | Computer: {ComputerScore}")
print("===============================")
print("")
if NumberOfRounds==5:
gameOn=False
break
if PlayerScore==ComputerScore:
print("Draw!!")
elif PlayerScore>ComputerScore:
print(f"Congrats {name.title()}, You won the game!!")
else:
print(f"Oops Computer won the game!! Better luck next time {name.title()}!")
Let’s play Rock, Paper or scissors with Coding!