What is a Dictionary?
Do you know the meaning of “Serendipity”? If you don’t – you would quickly want to search for it on the internet. Think of the times when there was no internet what did we do then …. “Dictionaries”. A book where words are paired with their meanings.
A python dictionary is similar. It is a data structure when you want to work with data collection. It’s an ordered (as of python 3.7) key-value pair which is mutable. It allows post-creation changes to the elements but is close to duplication, thus key/value pair should be unique. Like an English dictionary were to look up a meaning we search through the word, in a python dictionary to find a value we locate it using the key.
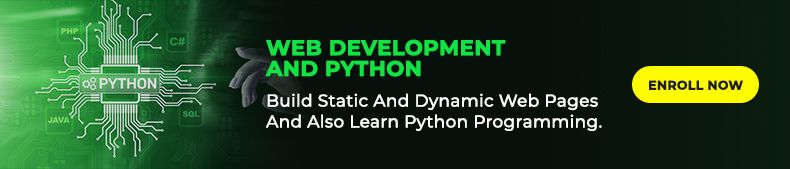
Creating a Dictionary
Creating a dictionary is very simple. We start by declaring a variable and using the assignment operator; on the right, we assign the data. The kind of brackets used is the significant factor that makes it a dictionary. To create a dictionary we use curly brackets { }.
Dict={ }
Would create an empty dictionary. In this method, the items/elements are added using one of the approaches mentioned below.
Or we can create a dictionary with two elements of key-value pair as stationery items
Dict={“pen”:”Gel”,”pencil”:”color”,”marker”: “Black”}
Accessing a Dictionary
There are many ways we can access items of a Python dictionary. Python has built-in methods to interact with dictionaries. The easiest way is by referring to its key inside the square brackets.
The bracket notation is best used when we want to access a single item in the dictionary. For example, to get the type of pencil, we can code as follows:
print(Dict[“pencil”])
Get(): Another way to access an item is the get method. Similar to the bracket notation the get function fetches the value based on the key passed.
X=Dict.get(“pencil”)
Keys(): The keys method can be used to retrieve all the keys of the collection. Running this code, the dictionary will return all the keys within it.
X=Dict.keys()
Print(X)
Values(): This method is similar to the keys function. The difference is that the values method would return the current values in the dictionary instead of keys. This can be useful if want to see just the values to locate particular values to be modified.
X=Dict.values()
Print(X)
Items(): The items method of python returns the full dictionary as key-value pair.
X=Dict.items()
Print(X)
Adding Elements
Dictionaries can be modified even after creating them by adding new entries to them. This gives power to the programmer so that he can grow the dictionary which facilitates its long-term use.
The use of the square bracket notion – one adds an element to the dictionaries by stating the new key as the index. Assigning this key to a value inserts the pair in the previously created dictionary. Let’s understand how to do this through our example of a stationery dictionary.
Dict={
“pen”:”Gel”,
“pencil”:”color”,
“marker”:”black”
}
Dict[“highlighter”]=”Yellow” Adds a new element
Another manner to add a pair or update the existing one is by using the update function. The update method updates the existing if it’s already there in the dictionary or adds the key/value pair together if it is not present. The given example updates the marker key by changing its value from black to yellow.
Dict={
“pen”:”Gel”,
“pencil”:”color”,
“marker”:”black”
}
newDict.update({“marker”:”yellow”}) Updates marker element
Removing Elements
There is always need to remove elements from the collection when they are no longer needed. An element can be removed or deleted from the dictionary using any of the following techniques –
- Pop()– Using pop function we can remove an item by specifying the targeted key which allows us to restructure the dictionary as per the need.
Dict.pop(“pen”)
- Popitem()– This function has the limitation of not being able to specify which key to delete. The use of this function deletes the last item of the dictionary.
Dict.popitem()
- Del Keyword– This keyword is used in front of the dictionary call to specify the target key using the square brackets. The code displays how we can delete the key-value pair pen from the dictionary.
Del Dict[“pen”]
When the Del keyword is used just with dictionary call and without the square brackets it can be used to delete the entire dictionary. Such a thing is usually done when a student is no longer studying at school so we might want to remove all details.
Del Dict
- Clear()- Another method clear removes all the contents of the dictionary while leaving the dictionary object unharmed.
Dict.clear()
Nested Dictionaries
Dictionaries of python have the capability to have a dictionary inside a dictionary, it’s a level deeper concept for next time. For now, an example, if I am creating a dictionary of a family and there are two children so –
myfamily = {
"child1" : {
"name" : "Son1",
"year" : 2004
},
"child2" : {
"name" : "Son2",
"year" : 2007
},
Practical Use
Dictionaries are very convenient when trying to keep records of say Student’s grades. Using students as the key one can easily find details of grades etc. Another example can be while searching for a word frequency.
Often freshers in programming even while being well aware of dictionaries are seen as confused about where to use them. Thus, often a task that can be easily done using dictionaries they are founded trying to achieve through some other data structures maybe a list. At SkoolofCode while creating a strong base for its students to understand how to create, manipulate or delete dictionaries in python for kids. We also emphasize on the wide vision of the practical usage of this data structure.
What would you use – “List” or “Dictionary” when you want faster access while looking up an element?
Are you seeking introductory or advanced online coding classes for kids? At SkoolOfCode, we offer project-based learning modules where students use live code to find logical and inventive solutions to problems. So, why wait and Book a FREE trial class today?
By: Ms. Divya Dalal, an educator working with SkoolofCode taking Scratch and Python classes. She is an MCA with, a Master’s in Technology in Software Engineering.